UI
ListView
<ListView>
is a UI component that shows items in a vertically scrolling list. To set how the list shows individual items, you can use a template
component,e.g <ListView.itemTemplate>
for plain JS. Using a ListView requires some special attention due to the complexity of the native implementations, with custom item templates, bindings and so on.
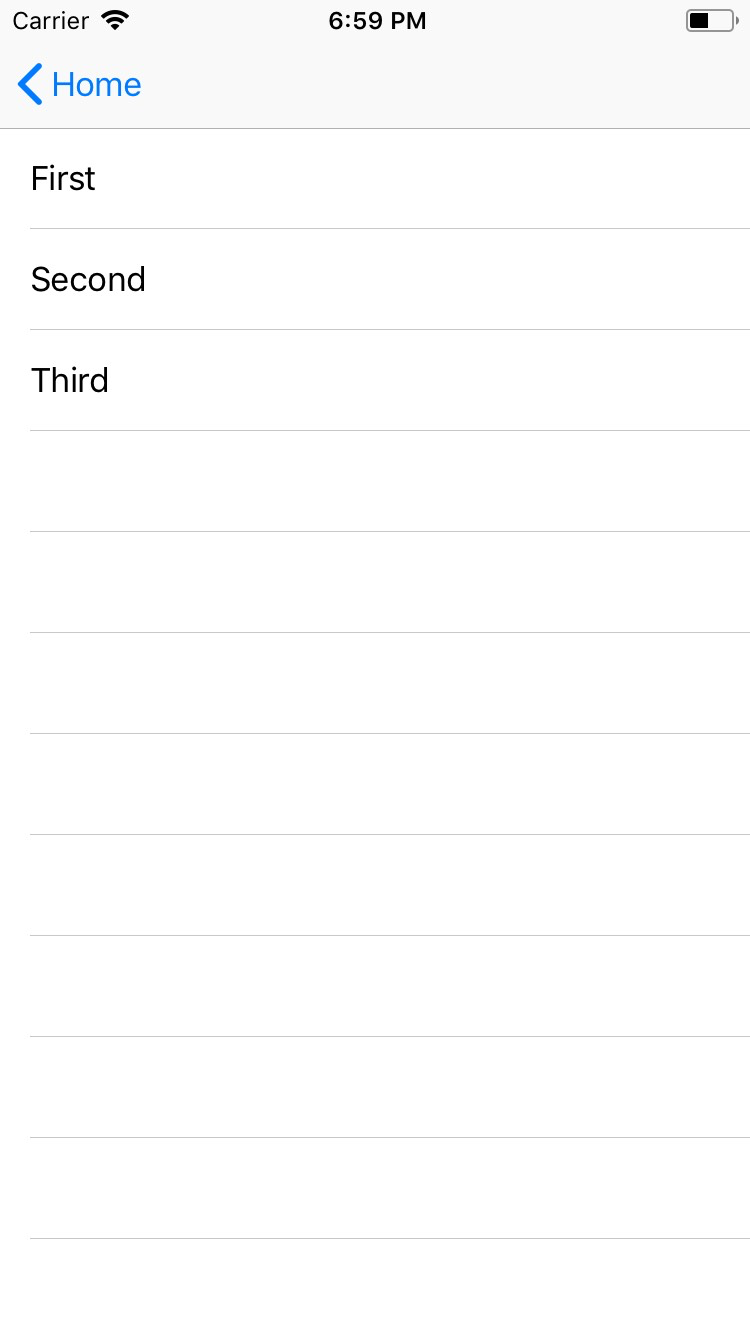
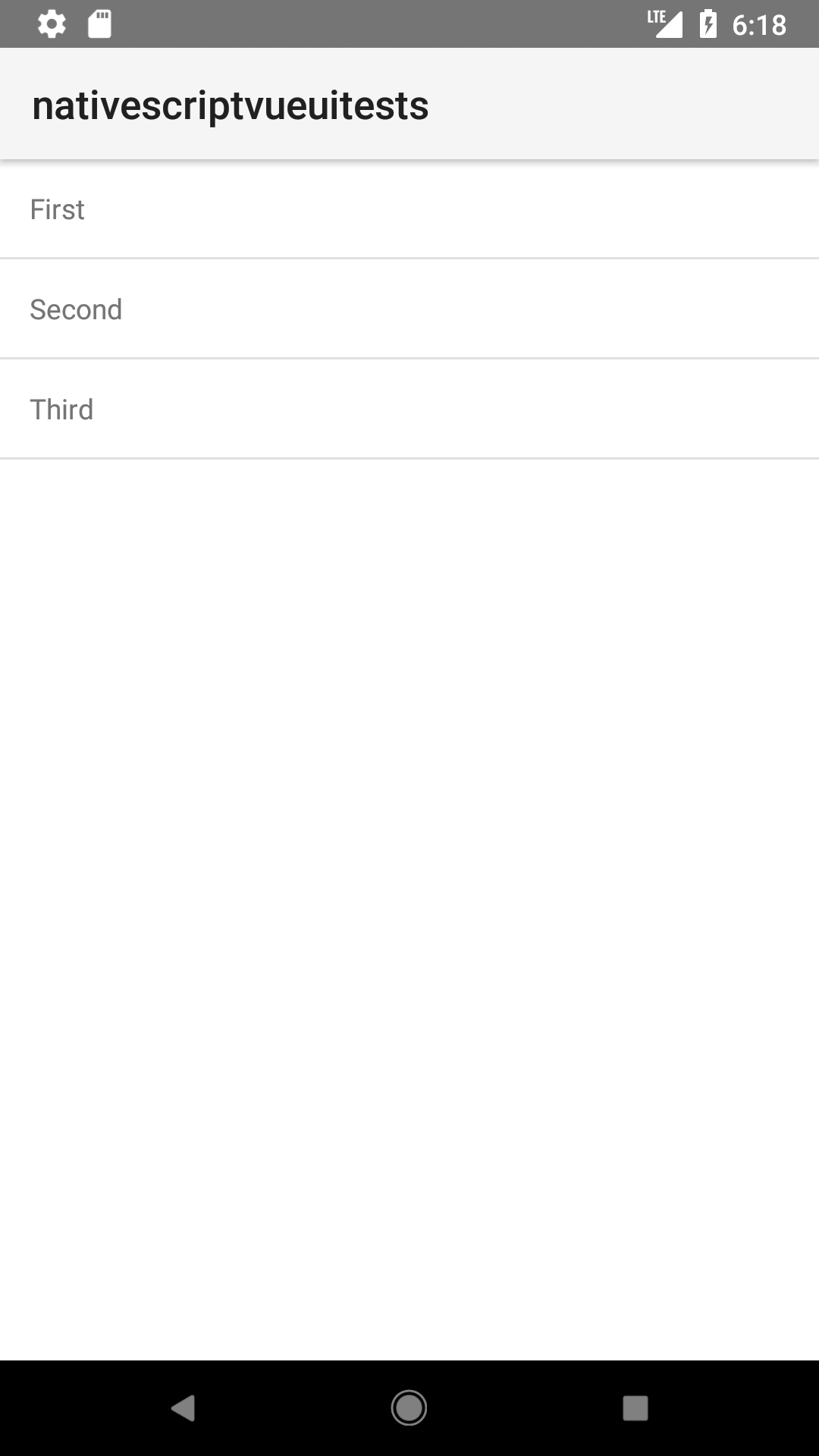
Creating a ListView
Note
The ListView's item template can contain only a single root view container.
<ListView
items="{{ titlesArray }}"
loaded="{{ onListViewLoaded }}"
itemTap="onItemTap"
loadMoreItems="onLoadMoreItems"
separatorColor="orangered"
rowHeight="50"
class="list-group"
id="listView"
>
<ListView.itemTemplate>
<!-- The item template can only have a single root view container (e.g. GriLayout, StackLayout, etc.) -->
<StackLayout class="list-group-item">
<Label text="{{ title || 'Downloading...' }}" textWrap="true" class="title" />
</StackLayout>
</ListView.itemTemplate>
</ListView>
import {
EventData,
fromObject,
ListView,
ObservableArray,
ItemEventData,
Page,
} from '@nativescript/core'
export function onNavigatingTo(args: EventData) {
const page = args.object as Page
const titlesArray = new ObservableArray([
{ title: 'The Da Vinci Code' },
{ title: 'Harry Potter and the Chamber of Secrets' },
{ title: 'The Alchemist' },
{ title: 'The Godfather' },
{ title: 'Goodnight Moon' },
{ title: 'The Hobbit' },
])
const vm = Observable()
vm.titlesArray = titlesArray
page.bindingContext = vm
}
export function onListViewLoaded(args: EventData) {
const listView = args.object as ListView
}
export function onItemTap(args: ItemEventData) {
const index = args.index
console.log(`Second ListView item tap ${index}`)
}
export function onLoadMoreItems(args: ItemEventData) {
if (loadMore) {
console.log('ListView -> LoadMoreItemsEvent')
setTimeout(() => {
listArray.push(
moreListItems.getItem(Math.floor(Math.random() * moreListItems.length))
)
listArray.push(
moreListItems.getItem(Math.floor(Math.random() * moreListItems.length))
)
listArray.push(
moreListItems.getItem(Math.floor(Math.random() * moreListItems.length))
)
listArray.push(
moreListItems.getItem(Math.floor(Math.random() * moreListItems.length))
)
listArray.push(
moreListItems.getItem(Math.floor(Math.random() * moreListItems.length))
)
}, 3000)
loadMore = false
}
}
Props
items
<ListView items="{{ years }}">
Gets or set the items collection of the ListView
. The items property can be set to an array or an object defining length and getItem(index) method.
itemTemplateSelector
A function that returns the appropriate keyed template based on the data item.
itemTemplates
Gets or set the list of item templates for the item template selector.
separatorColor
<ListView items="{{ titlesArray }}" separatorColor="orangered">
Gets or set the color of the separator line for the ListView instance.
rowHeight
<ListView items="{{ titlesArray }}" rowHeight="50">
Gets or set row height of the ListView.
iosEstimatedRowHeight
Gets or set the estimated height of rows in the ListView. Default value: 44px
Methods
refresh()
listView.refresh()
Forces the ListView to reload all its items.
scrollToIndex()
listView.scrollToIndex(index: number)
Scrolls the item with the specified index into view.
scrollToIndexAnimated()
listView.scrollToIndexAnimated(index: number)
Scrolls the item with the specified index into view with animation.
isItemAtIndexVisible()
isItemVisible: boolean = listView.isItemAtIndexVisible(index: number)
Checks if the item with the specified index is visible.
Event(s)
itemTap
onListViewLoaded(args: EventData) {
const listView = args.object as ListView
listView.on("itemTap", (itemTapData: ItemEventData) => {
console.log(Object.keys(itemTapData))
})
}
The event is raised when an item inside the ListView is tapped. See ItemEventData interface for the event data.
itemsLoading
onListViewLoaded(args: EventData) {
const listView = args.object as ListView
listView.on("itemsLoading", (itemsLoadingData: ItemEventData) => {
})
}
Visit ItemsLoading interface for the event data.
loadMoreItems
The event will be raised when the ListView is scrolled so that the last item is visible. It is intended to be used to add additional data in the ListView.
Native component
Android | iOS |
---|---|
android.widget.ListView | UITableView |
- Previous
- ListPicker
- Next
- Placeholder