Note: You are on the beta version of our docs. This is a work in progress and may contain broken links and pages.
UI
Progress
<Progress>
is a UI component that shows a bar to indicate the progress of a task.
See also: ActivityIndicator.
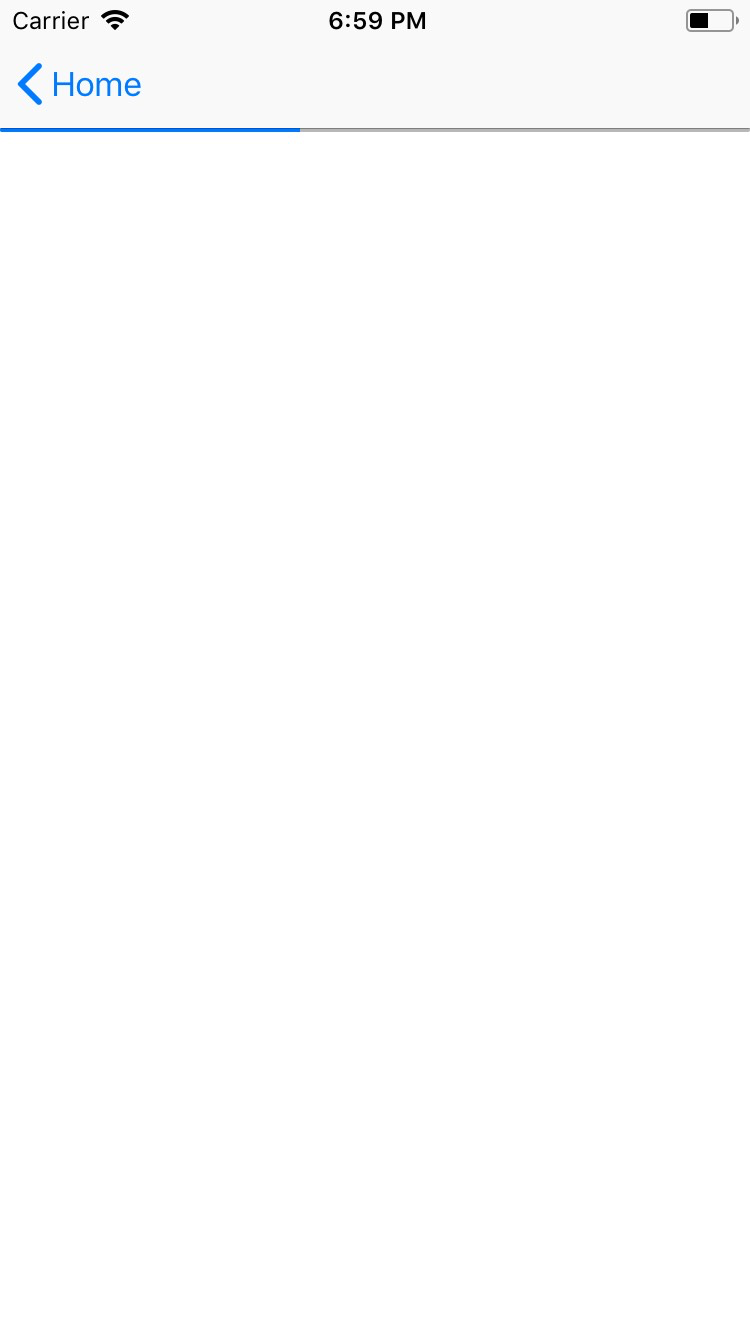
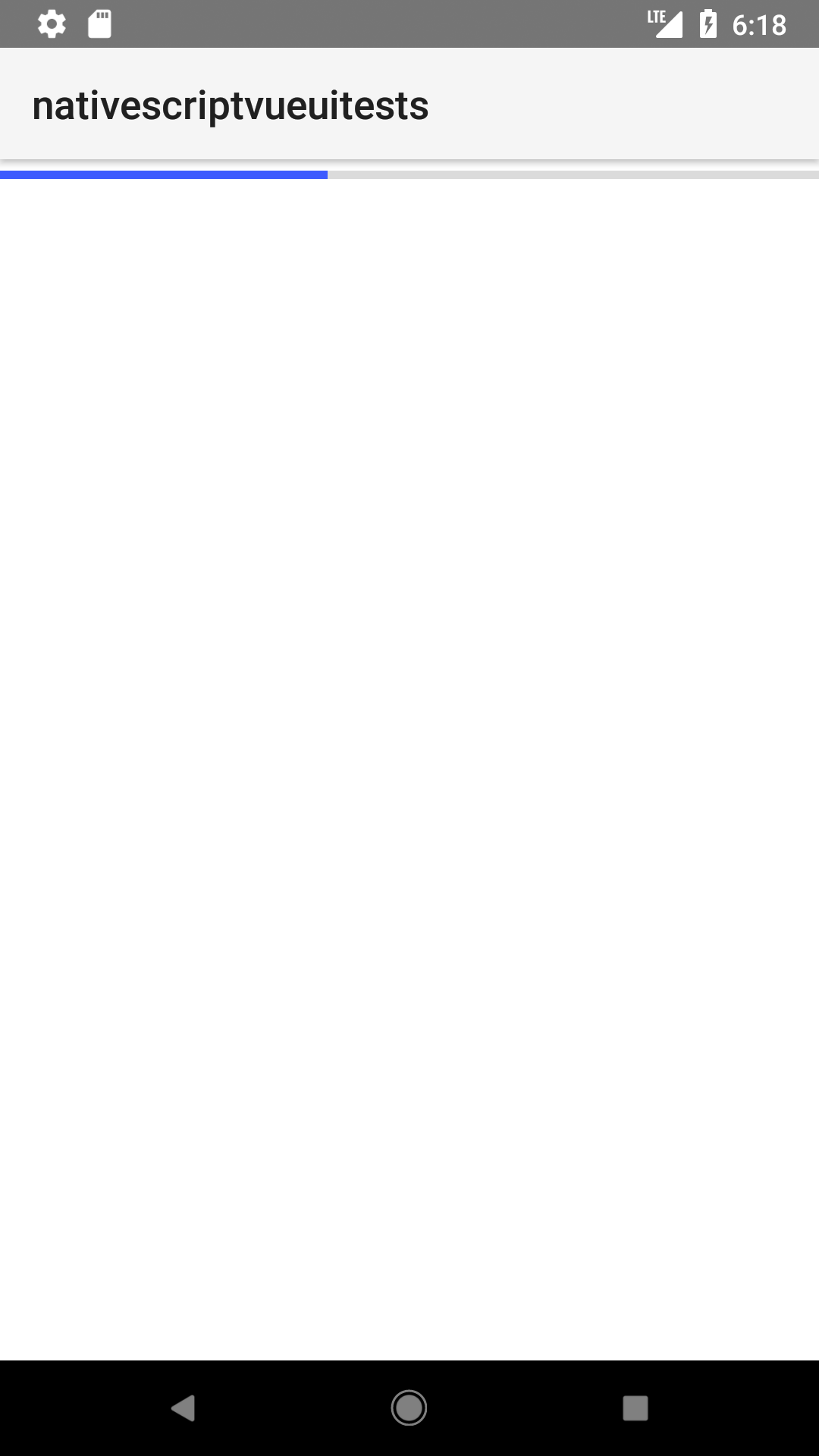
Creating a Progress
xml
<Progress
width="100%"
value="{{ progressValue }}"
maxValue="{{ progressMaxValue }}"
loaded="onProgressLoaded"
/>
ts
import {
Observable,
Page,
Progress,
PropertyChangeData,
} from '@nativescript/core'
export function onNavigatingTo(args) {
const page = args.object as Page
vm.set('progressValue', 10) // Initial value
vm.set('progressMaxValue', 100) // Maximum value
// Forcing progress value change (for demonstration)
setInterval(() => {
const value = vm.get('progressValue')
vm.set('progressValue', value + 2)
}, 300)
page.bindingContext = vm
}
export function onProgressLoaded(args) {
const myProgressBar = args.object as Progress
myProgressBar.on('valueChange', (pargs: PropertyChangeData) => {
// TIP: args (for valueChange of Progress) is extending EventData with oldValue & value parameters
console.log(`Old Value: ${pargs.oldValue}`)
console.log(`New Value: ${pargs.value}`)
})
}
Styling Progress
Using backgroundColor
(CSS: background-color
) & color to change the Progress style.
Note
backgroundColor
will work only on iOS
; on Android
the background will be the color with applied opacity.
xml
<progress
value="50"
maxValue="100"
backgroundColor="red"
color="green"
></progress>
<!-- Using @nativescript/tailwind to change the Progress style -->
<progress value="25" maxValue="100" class="bg-red-500 text-red-900"></progress>
Props
value
xml
<Progress value="{{ progressValue }}" />
ets or sets the current value of the progress bar. Must be within the range of 0 to maxValue.
maxValue
xml
<Progress maxValue="{{ progressMaxValue }}" />
Gets or sets the maximum value of the progress bar. Defaults to: 100
.
...Inherited
For additional inherited properties, refer to the API Reference
Events
valueChange
Emitted when the value
property changes.
Native Component
Android | iOS |
---|---|
android.widget.ProgressBar (indeterminate = false) | UIProgressView |
- Previous
- Placeholder
- Next
- ScrollView