Note: You are on the beta version of our docs. This is a work in progress and may contain broken links and pages.
UI
Slider
<Slider>
is a UI component that provides a slider control for picking values within a specified numeric range.
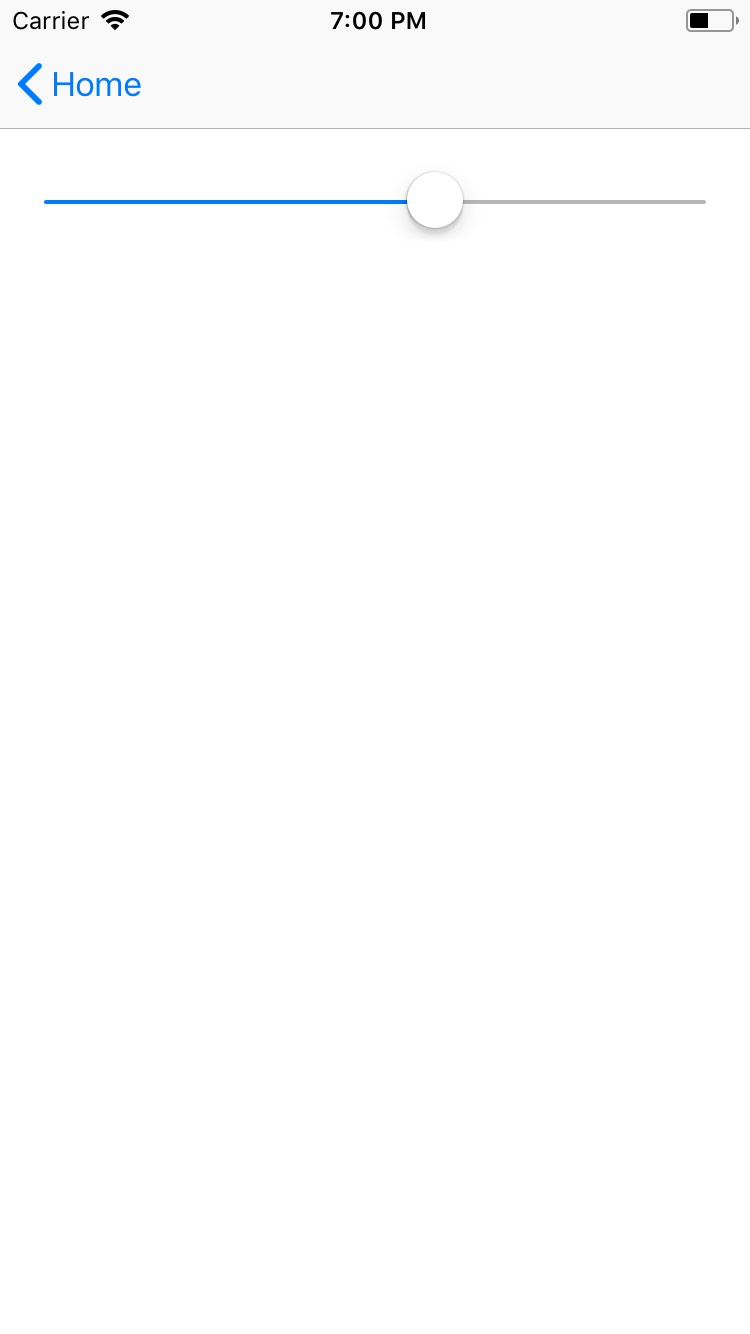
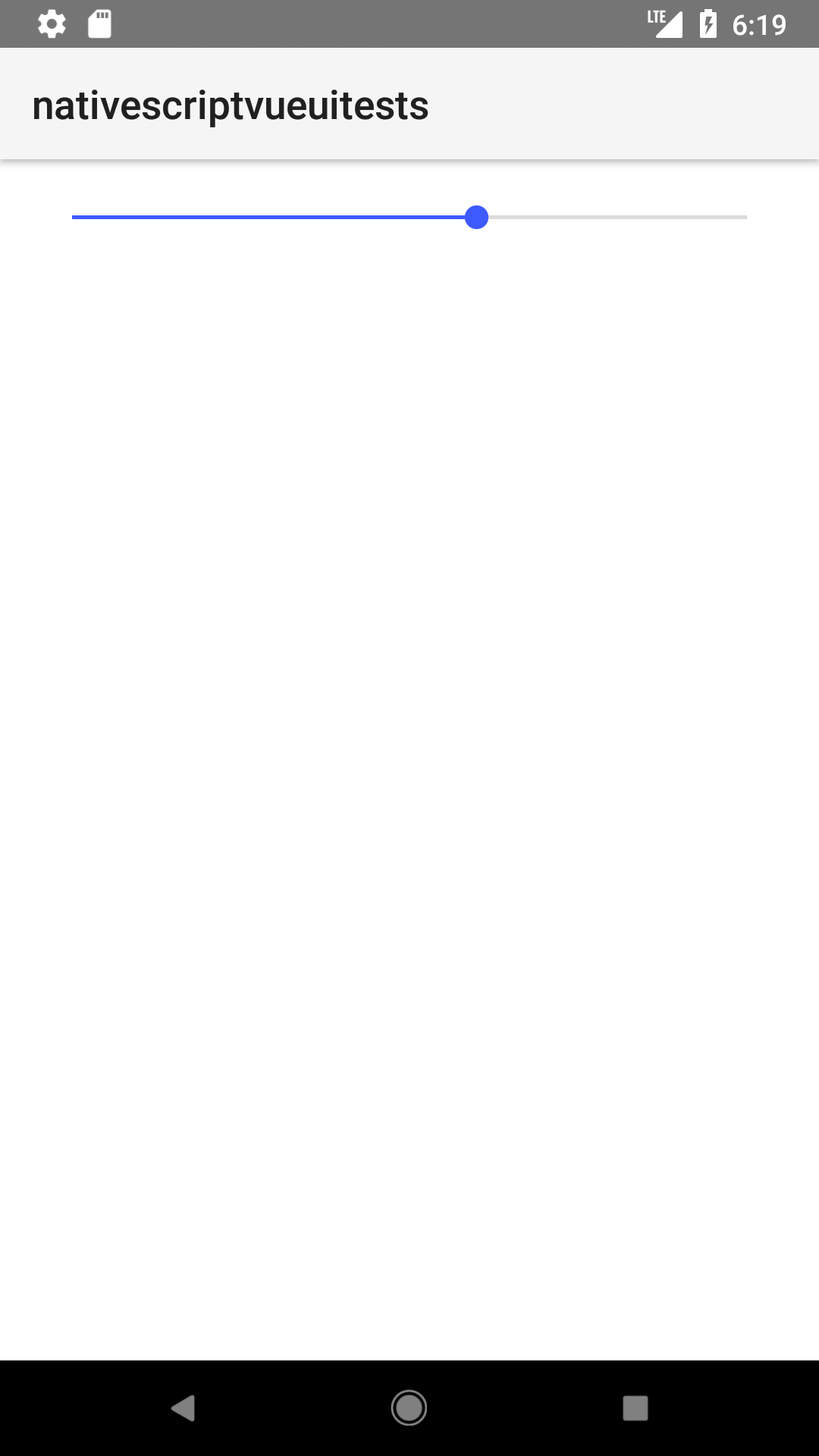
Simple Slider and listening to its value change event
To listen to the valueChange
event, register the listener in the Slider's loaded
event handler.
xml
<Slider value="10" minValue="0" maxValue="100" loaded="{{ onSliderLoaded }}" />
ts
import { Observable, Slider } from '@nativescript/core'
export class HelloWorldModel extends Observable {
constructor() {
super()
}
onSliderLoaded(args: EventData) {
const slider = args.object as Slider
slider.on('valueChange', (args: PropertyChangeData) => {
console.log('valueChange: ', args.value, 'Old: ' + args.oldValue)
})
}
}
Props
value
xml
<Slider value="10" />
Gets or sets the currently selected value of the slider. Defaults to 0
.
minValue
xml
<Slider minValue="0" />
Gets or sets the minimum value of the slider.
maxValue
xml
<Slider maxValue="100"/>
Gets or sets the maximum value of the slider.
...Inherited
For additional inherited properties, refer to the API Reference.
Event(s)
valueChange
ts
slider.on('valueChange', (args: PropertyChangeData) => {
console.log('valueChange: ', args.value, 'Old: ' + args.oldValue)
})
Emitted when the value of the slider changes. See PropertyChangeData interface for available data.
Native component
Android | iOS |
---|---|
android.widget.SeekBar | UISlider |
- Previous
- SegmentedBar
- Next
- Switch